반응형
4. Multivariable Linear regression(다항회귀분석)
- Simple Linear REgression 복습
- Multivariate Linear Regression 이론
- Naive Data Representation
- Matrix Data Representation
- Multivariate Linear Regression
- nn.Module 소개
- F.mse_loss 소개
Simple Linear Regrssion?
- 하나의 정보로부터 하나의 결론을 짓는 모델
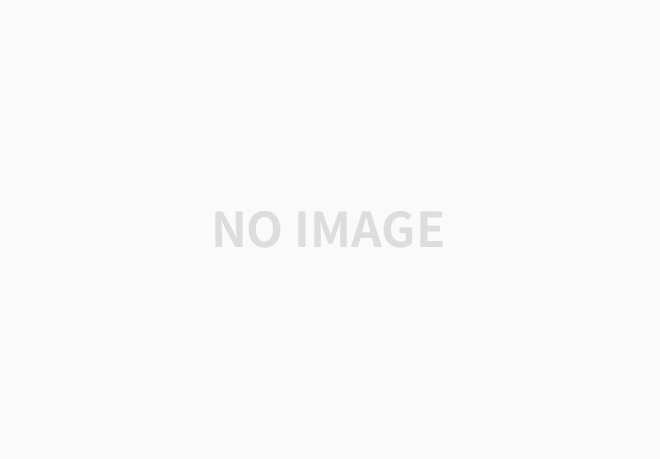
- ${H(x) = Wx + b}$
그러나 우리가 더 다양한 정보를 가지고 다양한 예측을 하기위해서는 단항선형회귀분석으로는 어렵다!
Multivariate Linear Regression
- 복수의 정보가 존재할 떄 하나의 추측값을 계산하는 것
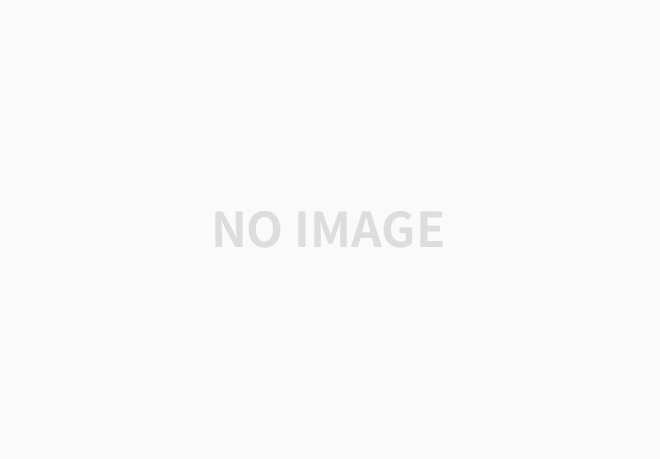
실습
DATA
다섯명의 학생이 세번의 쪽지시험에서 받은 점수들과 기말고사 점수(y) 를 가지고 쪽지시험의 점수를 받았을 때 기말고사 점수를 예측하는 과정
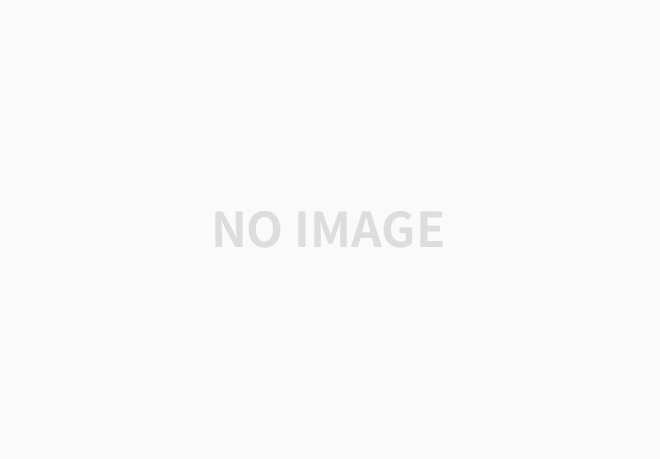
Hypothesis Function
$${H(x) = Wx + b}$$
- x라는 vector와 W라는 matrix의 곱
$${H(x) = w_1x_1 + w_2x_2 + w_3x_3 + b}$$
- 입력 변수가 3개면 weight도 3개
Hypothesis Fuction : Naive
- 단순한 hypothesis 정의!
- ${H(x) = w_1x_1 + w_2x_2 + w_3x_3 + b}$
- 하지만 x가 길이 1000의 vector라면?
Hypothesis Function : Matrix
- matmul()로 한번에 계산
- 더 간결하고, x의 길이가 바뀌어도 코드를 바꿀 필요가 없으며, 속도도 더 빠르다!
- 𝐻(𝑥)=𝑊𝑥+𝑏
Cost function : MSE
- 기존 Simple Linear Regression과 동일한 공식!
- 예측값과 실제값의 차이를 제곱한 평균
- ${cost(W) = \frac{1}{m} \sum_{i = 1}^{m} (H(x^{(i)}) - y^{(i)})^2}$
Gradient Descent with torch.optim
$${\nabla{W} = \frac{\partial{cost}}{\partial{W}} = \frac{2}{m}\sum_{i=1}^{m}(Wx^{(i)} - y^{(i)})x^{(i)}}$$
$${W : = W - \alpha\nabla{W}}$$
Full Code with torch.optim
import torch
from torch import optim
## 데이터
x_train = torch.FloatTensor([[73, 80, 75],
[93, 88, 93],
[89, 91, 90],
[96, 98, 100],
[73, 66, 70]])
y_train = torch.FloatTensor([[152], [185], [180], [196], [142]])
# 모델 초기화
W = torch.zeros((3, 1), requires_grad = True)
b = torch.zeros(1, requires_grad = True)
# optimizer 설정
optimizer = optim.SGD([W, b], lr =1e-5)
nb_epochs = 20
for epoch in range(nb_epochs + 1):
# H(x) 계산
hypothesis = x_train.matmul(W) + b
# cost 계산
cost = torch.mean((hypothesis - y_train)**2)
# cost로 H(x) 계산
optimizer.zero_grad()
cost.backward()
optimizer.step()
print('Epoch {:4d}/{} hypothesis: {} Cost : {:.6f}'.format(
epoch, nb_epochs, hypothesis.squeeze().detach(), cost.item()
))
>>>>
Epoch 0/20 hypothesis: tensor([0., 0., 0., 0., 0.]) Cost : 29661.800781
Epoch 1/20 hypothesis: tensor([67.2578, 80.8397, 79.6523, 86.7394, 61.6605]) Cost : 9298.520508
Epoch 2/20 hypothesis: tensor([104.9128, 126.0990, 124.2466, 135.3015, 96.1821]) Cost : 2915.712646
Epoch 3/20 hypothesis: tensor([125.9942, 151.4381, 149.2133, 162.4896, 115.5097]) Cost : 915.040527
...
Epoch 19/20 hypothesis: tensor([152.8014, 183.6715, 180.9666, 197.0686, 140.0985]) Cost : 1.619770
Epoch 20/20 hypothesis: tensor([152.8020, 183.6731, 180.9677, 197.0699, 140.1000]) Cost : 1.619033
- epoch를 반복할수록 점점 cost가 작아지고 H(x)가 y에 가까워진다.
- Learning rate에 따라서 발산할 수도 있음
nn.Module
-
W와 b를 일일히 쓰는 것은 모델이 커질수록 귀찮은 일이 될 수 있다.
-
nn.Module을 상속해서 모델 생성
-
nn.Linear(3, 1)
- 입력 차원 : 3
- 출력 차원 : 1
-
Hypothesis 계산은 forward()에서
-
Gradient 계산은 Pytorch가 알아서 해준다 backward()
import torch.nn as nn
class MultivariateLinearRegressionModel(nn.Module):
def __init__(self):
super().__init__()
self.linear = nn.Linear(3, 1) # 입력차원(W의 길이), 출력차원(y의 값)
def forward(self, x):
return self.linear(x)
# hypothesis = model(x_train)
F.mse_loss
- torch.nn.functional에서 제공하는 loss function 사용
- 쉽게 다른 loss와 교체 가능(l1_loss, smoth_l1_loss 등...)
import torch.nn.functional as F
# cost 계산
# cost = F.mse_loss(prediction, y_train)
Full Code with torch.optim
## 데이터
x_train = torch.FloatTensor([[73, 80, 75],
[93, 88, 93],
[89, 91, 90],
[96, 98, 100],
[73, 66, 70]])
y_train = torch.FloatTensor([[152], [185], [180], [196], [142]])
# 모델 초기화
# W = torch.zeros((3, 1), requires_grad = True)
# b = torch.zeros(1, requires_grad = True)
model = MultivariateLinearRegressionModel()
# optimizer 설정
optimizer = optim.SGD(model.parameters(), lr =1e-5)
nb_epochs = 20
for epoch in range(nb_epochs + 1):
# H(x) 계산
# hypothesis = x_train.matmul(W) + b
hypothesis = model(x_train)
# cost 계산
# cost = torch.mean((hypothesis - y_train)**2)
cost = F.mse_loss(hypothesis, y_train)
# cost로 H(x) 계산
optimizer.zero_grad()
cost.backward()
optimizer.step()
print('Epoch {:4d}/{} hypothesis: {} Cost : {:.6f}'.format(
epoch, nb_epochs, hypothesis.squeeze().detach(), cost.item()
))
>>>
Epoch 0/20 hypothesis: tensor([37.7348, 43.9963, 44.0900, 46.9199, 34.2331]) Cost : 17049.736328
Epoch 1/20 hypothesis: tensor([ 88.7252, 105.2839, 104.4771, 112.6801, 80.9802]) Cost : 5345.532715
Epoch 2/20 hypothesis: tensor([117.2726, 139.5968, 138.2856, 149.4968, 107.1525]) Cost : 1676.886353
...
Epoch 19/20 hypothesis: tensor([153.5754, 183.2467, 181.2860, 196.3242, 140.4497]) Cost : 1.943603
Epoch 20/20 hypothesis: tensor([153.5757, 183.2481, 181.2868, 196.3252, 140.4509]) Cost : 1.942654
출처 : www.boostcourse.org/ai214/lecture/42286
파이토치로 시작하는 딥러닝 기초
부스트코스 무료 강의
www.boostcourse.org
반응형
'AI Study > DL_Basic' 카테고리의 다른 글
[파이토치로 시작하는 딥러닝 기초]05_ Logistic Regression (0) | 2020.12.22 |
---|---|
[파이토치로 시작하는 딥러닝 기초]04.02_Loading Data (0) | 2020.12.21 |
[파이토치로 시작하는 딥러닝 기초]03_Deeper Look at Gradient Descent (0) | 2020.12.21 |
[파이토치로 시작하는 딥러닝 기초]02_Linear Regression (0) | 2020.12.21 |
[파이토치로 시작하는 딥러닝 기초]01_Tensor Manipulation(텐서 조작) (0) | 2020.11.26 |